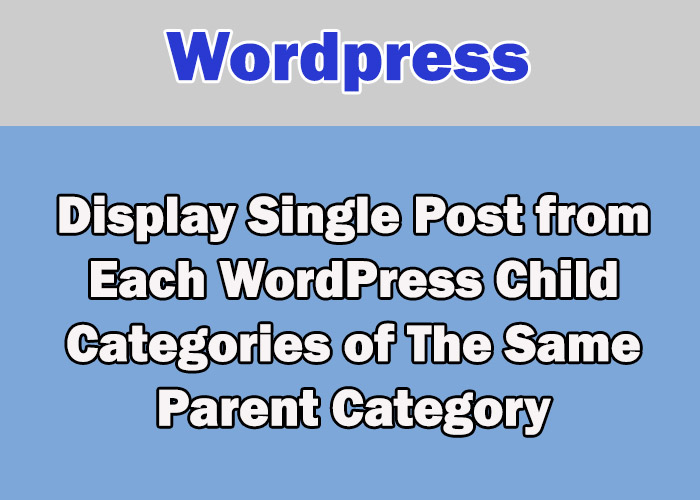
I hope your search will end here because I am going to share and explain tested WordPress code that will help you in retrieving/displaying single WordPress posts from each WordPress child category having the same parent category in WordPress. Although WordPress functions are well documented but not every time our desired functionality can be achieved just by using WordPress existing functions.
One of my client asked for developing a page which will display one post from each child category of a specific parent category, I agreed and was happy for this simple task, which I thought would take me 30 at maximum for implementing this functionality. It took me one hour and 30 minutes (testing included) because of figuring out a way for achieving the desired result with the help of WordPress existing functions, I searched for a quick solution but found none, that’s why I developed my own solution and am sharing with you to save your time for development.
The first solution which came to my mind was using query_posts(), it did displayed posts form the specified parent category but multiple posts from some child categories in which i had recently posted. i would say that query_posts() does not guarantee displaying one post from each child category. For example if you have 9 child categories under a parent category let say “Tutorials”.
Tutorials (parent category)
- php
- wordpress
- html
- laraval
- css
- javascript
- jQuery
- Java
- c#
When we use query_posts(id of Tutorials) parent category for displaying only nine posts from unique child category, it will display nine posts but it is not guaranteed that they will be from unique child categories, in-fact query_posts() will display nine recent posts having the specified parent category. If you have recently posted 5 posts in “php” category then all five of them will be displayed first.
In this tutorial I have used only three WordPress functions with some conditional checks.
Assuming that you are familiar with the use of above wordpress functions, I will directly go to the solution, each line of the code below has explanatory comments. You can use this code multiple times without querying the database multiple times, just read the code explanation section below.
<?php
$args = array('posts_per_page' => -1); // we want to fetch all posts
$my_posts = new WP_Query($args);
$postsFlag = 0; // this variable will limit the number of post you want to display
$tutsPosts = array(); // this array will hold ID's of child categories
$tutsTerm=get_term_by( 'name', 'Tutorials', 'category' ); // get term object of tutorials, here Tuutorials is the parent category.
if ($my_posts->have_posts()) : while ($my_posts->have_posts()) : $my_posts->the_post();
$cats= get_the_category(); // get categories of current post
$currentCat = get_term_by( 'name', $cats[0]->name, 'category' ); // get term object of 1st category
if($tutsTerm->term_id==$currentCat->parent){ // check if parent of current category == tutorials
if($postsFlag==0){ // postsFlag will return only 9 posts
$tutsPosts[$postsFlag]=$currentCat->term_id; /*save id of current term to tutsPosts array, this array will contain single unique posts from each child category of Tutorials */
?>
<a href="<?php the_permalink();?>" ><?php the_title();?></a>
<?php $postsFlag++;
} // end of if postsFlag==0
else if($postsFlag<9){
$arrlength = count($tutsPosts);
$dupCount=0;
for($x = 0; $x < $arrlength; $x++) { // this loop will check for duplicate child catgories
if($tutsPosts[$x]==$currentCat->term_id){
$dupCount=1;
}
}// for loop end
if($dupCount==0){
$tutsPosts[$postsFlag]=$currentCat->term_id;
?>
<a href="<?php the_permalink();?>" ><?php the_title();?></a>
<?php
$postsFlag++;
} // end of duplicate check
} // if postFlag < 9 check end
else{ // break the loop
break;
} // end of postFlag check
}// end of check if parent of current category == tutorials
?>
<?php endwhile; endif; ?>
Code Explanation:
$args = array('posts_per_page' => -1); // we want to fetch all posts
$my_posts = new WP_Query($args);
As i am using this code in a separate page template hence running a custom wordpress loop using new WP_Query($args), through $args (arguments) variable i am telling wordpress to fetch all posts from the database. Fetching all posts from wordpress database is useful if you don’t want to run multiple queries for multiple wordpress loops, you just have to use $myposts->rewind_posts() each time before starting a a new posts loop. This way querying database multiple times can be avoided. If you are going to display posts from only one parent category then you can limit the WP_Query($args) to that category only. If you are going to use this code multiple times then do not use the above lines of code again, all you have to do is call the $my_posts ->rewind_posts() before starting a new loop and you are all set.
Replace “
$args = array(‘posts_per_page’ => -1);
$my_posts = new WP_Query($args);
” with This “$my_posts->rewind_posts();“
$tutsPosts = array(); // this array will hold ID's of child categories
we will save id’s of every child category to $tutsPosts array for avoiding duplicate posts from the same child category.
$tutsTerm=get_term_by( 'name', 'Tutorials', 'category' ); // get term object of tutorials, here Tutorials is the parent category.
$tutsTerm will contain the id of Tutorials as this is the parent category which we will check for each post in the loop. If the post parent category id == tutorials id then that post will be displayed and its category id will be added to the $tutsPosts array.
$cats= get_the_category(); // get categories of current post
$currentCat = get_term_by( 'name', $cats[0]->name, 'category' ); // get term object of 1st category
get_the_category() returns all the categories assigned to a post, here we are accessing 1st category assigned to the posts through $cats[0]->name, $currentCat will host object of the category to which current post is assigned.
if($tutsTerm->term_id==$currentCat->parent)
this will check if the parent category id of current child category is equal to tutorials.. I believe rest of the code is self explanatory and if you do require assistance do ask in comments i will gladly help you..
Thanks for this. I was looking for code.
Hello my name is Saeed and I’m reading this topic from Iran … Thank you very much for the usefulness