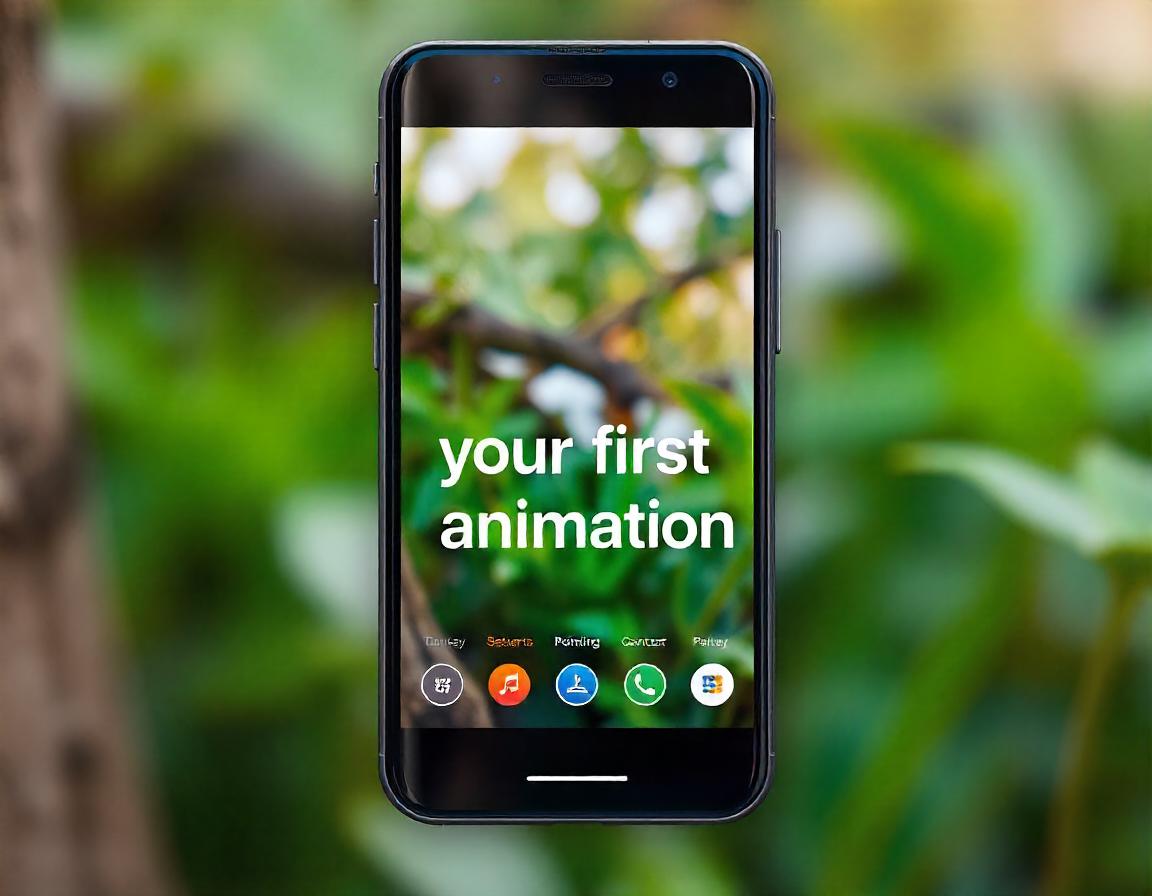
In the dynamic world of Android development, animations aren’t just eye candy—they’re the bridge between functionality and user experience. When used effectively, animations can make your app intuitive, engaging, and memorable. But where do you start, and what are the best techniques for creating animations in Android? Let’s embark on this journey together and unleash the power of motion in your apps!
Why Animations Matter in Android Apps
Imagine opening an app where buttons slide in seamlessly, images transform beautifully, or pages fade elegantly into one another. It feels professional, polished, and inviting, doesn’t it? That’s the magic of animations.
Animations help:
- Guide users through transitions.
- Provide feedback on interactions.
- Enhance the storytelling aspect of your app.
- Boost engagement and retention.
If you’ve never created animations before, don’t worry—this guide is here to empower you every step of the way to create animations.
Getting Started with Your First Animation
Before diving into advanced techniques, let’s start small. One of the simplest ways to implement an animation in Android is by using ObjectAnimator.
Step 1: Set Up Your Environment
Ensure you have Android Studio installed and ready. Create a new project or open an existing one where you want to add animations.
Step 2: Create a Simple Layout
Start with a simple XML layout. For example:
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<Button
android:id="@+id/animateButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"/>
</LinearLayout>
Step 3: Implement ObjectAnimator
ObjectAnimator lets you animate specific properties of a View. Here’s how you can make the button slide to the right:
kotlin
val button = findViewById<Button>(R.id.animateButton)
button.setOnClickListener {
val animator = ObjectAnimator.ofFloat(button, "translationX", 300f)
animator.duration = 1000 // 1 second
animator.start()
}
Congratulations! You’ve just created your first animation. Simple, right? Now let’s explore all the animation techniques Android offers.
Techniques for Animations in Android
Android provides a rich set of APIs and tools for creating animations. Each technique serves specific purposes and suits particular use cases.
1. Property Animations
- Introduced in Android 3.0 (API 11), Property Animations are the most versatile animation system. They allow you to animate any property of an object, such as size, position, rotation, or transparency.
- How to Use:
ObjectAnimator
,ValueAnimator
, andAnimatorSet
. - Best Use Cases:
- Moving objects across the screen.
- Creating fade-in or fade-out effects.
- Animating object rotation or scaling.
2. View Animations
- The classic animation system, also known as Tween Animation, lets you animate Views with predefined transformations like translation, rotation, scaling, or alpha.
- How to Use: XML or programmatically using
Animation
classes. - Best Use Cases:
- Animating entry and exit transitions.
- Adding simple visual effects for buttons or icons.
Example (XML):
xml
<translate
android:fromXDelta="0"
android:toXDelta="100%"
android:duration="500"/>
3. Drawable Animations
- Also called Frame-by-Frame animations, this technique involves displaying a sequence of images in rapid succession.
- How to Use: XML or
AnimationDrawable
. - Best Use Cases:
- Animating loading indicators.
- Creating playful or cartoon-like effects.
Example:
xml
<animation-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/frame1" android:duration="50" />
<item android:drawable="@drawable/frame2" android:duration="50" />
</animation-list>
4. Motion Layout
- MotionLayout, part of the ConstraintLayout library, is the most modern and powerful animation system for complex transitions and interactions.
- How to Use: Define animations in XML with a
MotionScene
. - Best Use Cases:
- Coordinating animations between multiple Views.
- Implementing swipe-based interactions.
Example:
<MotionScene>
<!-- Define transitions and keyframes here -->
</MotionScene>
5. Physics-Based Animations
- Introduced in Android 8.0 (API 26), physics-based animations simulate real-world behavior using springs and fling gestures.
- How to Use:
SpringAnimation
andFlingAnimation
. - Best Use Cases:
- Animating bouncing effects.
- Adding drag-and-release behaviors.
Example:
kotlin
val springAnim = SpringAnimation(button, DynamicAnimation.TRANSLATION_Y, 0f)
springAnim.start()
6. Transition Animations
- These are prebuilt animations for Activity or Fragment transitions.
- How to Use: Use Transition APIs or override
Activity
transitions. - Best Use Cases:
- Enhancing screen navigation.
- Creating shared element animations.
7. Custom Render Animations
- For scenarios where predefined animations won’t suffice, you can manually draw animations using a
Canvas
or OpenGL. - How to Use: Extend
View
and override theonDraw
method. - Best Use Cases:
- Developing games or custom visualizations.
- Creating particle effects.
Choosing the Right Animation Technique
Here’s a quick guide to help you decide:
Technique Best For Example Use Case Property Animations General-purpose animations Button slides, fade-ins View Animations Simple effects Icon rotation, entry animations Drawable Animations Frame-based effects Loading indicators Motion Layout Complex transitions Onboarding screens, card swipes Physics-Based Animations Realistic interactions Drag-and-drop effects Transition Animations Activity/Fragment transitions Page navigation, shared element effects Custom Render Animations Unique, high-performance visuals Games, fireworks
Tips for Creating Stunning Animations
- Start Simple: Don’t overwhelm yourself. Begin with small, manageable animations.
- Keep Performance in Mind: Avoid overloading the UI thread.
- Make It Purposeful: Every animation should serve a functional or aesthetic purpose.
- Experiment and Iterate: Creativity thrives on exploration. Test various styles and effects.
- Engage Your Users: Create animations that feel natural and responsive.
Inspiration for Your Journey
Every master animator started with a single frame. The fact that you’re here, reading this, shows your passion for creating something extraordinary. Your first animation might feel basic, but it’s a stepping stone to crafting experiences that users will adore.
Remember, animations aren’t just technical implementations—they’re a form of storytelling. And like every good story, it takes time and practice to tell it well.
Creating animations in Android is an exciting and rewarding endeavor. Whether you’re animating a button, crafting a beautiful transition, or designing an entire motion-based interface, the possibilities are endless.
So, roll up your sleeves, open Android Studio, and start animating. Your journey to building mesmerizing Android apps begins now.
Hope that we have helped you in getting started with your android animation, kindly follow us on Facebook and share this post with in your circle.